PHP新手入门:构建你的第一个数据库驱动的Web应用程序
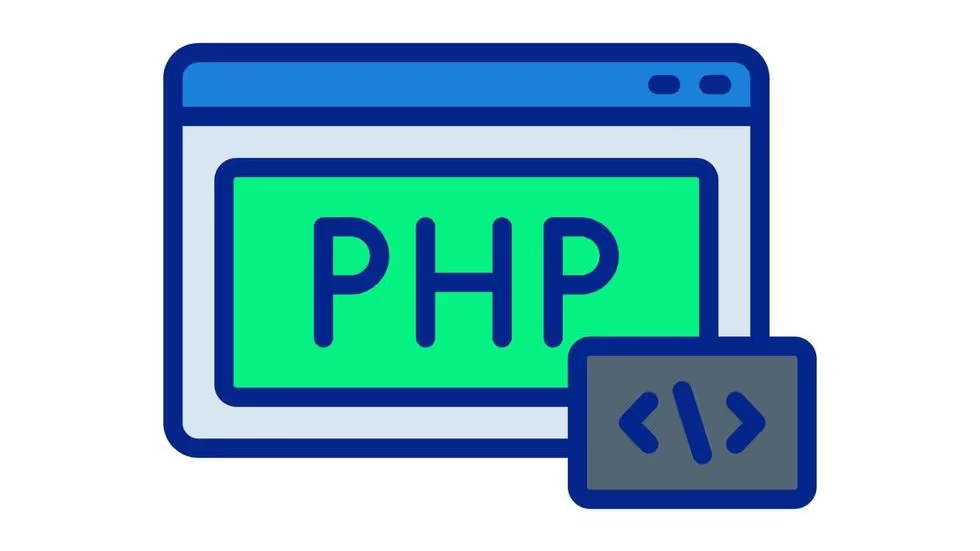
如果你刚开始学习PHP,那么构建一个数据库驱动的Web应用程序是你能着手进行的最令人兴奋的项目之一。这是了解后端如何工作、与数据库交互并为用户提供动态内容的绝佳方式。
编码起步
在本教程中,我们将使用PHP和MySQL构建一个简单的待办事项列表应用程序。完成后,你将拥有一个可运行的应用程序,用户可以在其中添加、查看和删除任务。
前提条件
在开始之前,请确保你具备以下条件:
- PHP(7.4版本或更高)
- MySQL(或MariaDB)
- 像XAMPP或Laragon这样的本地服务器
- 像VS Code这样的代码编辑器
步骤1:设置环境
- 安装本地服务器(例如XAMPP)。
- 启动Apache和MySQL服务。
- 导航到你的Web根目录(XAMPP的htdocs目录),并创建一个名为todo_app的新文件夹。
步骤2:创建数据库
- 打开phpMyAdmin。
- 创建一个名为todo_app的新数据库。
- 运行以下SQL查询来创建一个tasks表:
CREATE TABLE tasks (
id INT AUTO_INCREMENT PRIMARY KEY,
task VARCHAR(255) NOT NULL,
created_at TIMESTAMP DEFAULT CURRENT_TIMESTAMP
);
步骤3:构建HTML前端
在todo_app文件夹中创建一个index.php文件,并添加以下HTML代码:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>待办事项列表应用程序</title>
<link rel="stylesheet" href="styles.css">
</head>
<body>
<div class="container">
<h1>待办事项列表</h1>
<form action="add_task.php" method="POST">
<input type="text" name="task" placeholder="输入新任务" required>
<button type="submit">添加任务</button>
</form>
<ul>
<?php
// 从数据库中获取任务
$conn = new mysqli("localhost", "root", "", "todo_app");
$result = $conn->query("SELECT * FROM tasks ORDER BY created_at DESC");
while ($row = $result->fetch_assoc()) {
echo "<li>". $row['task'].
" <a href='delete_task.php?id=". $row['id']. "'>删除</a></li>";
}
$conn->close();
?>
</ul>
</div>
</body>
</html>
步骤4:处理添加任务
创建一个名为add_task.php的新文件,并添加以下代码:
<?php
if ($_SERVER['REQUEST_METHOD'] === 'POST') {
$task = $_POST['task'];
// 连接到数据库
$conn = new mysqli("localhost", "root", "", "todo_app");
// 将任务插入数据库
$stmt = $conn->prepare("INSERT INTO tasks (task) VALUES (?)");
$stmt->bind_param("s", $task);
$stmt->execute();
$stmt->close();
$conn->close();
// 重定向回主页面
header("Location: index.php");
exit();
}
?>
步骤5:处理删除任务
创建一个名为delete_task.php的新文件:
<?php
if (isset($_GET['id'])) {
$id = $_GET['id'];
// 连接到数据库
$conn = new mysqli("localhost", "root", "", "todo_app");
// 从数据库中删除任务
$stmt = $conn->prepare("DELETE FROM tasks WHERE id =?");
$stmt->bind_param("i", $id);
$stmt->execute();
$stmt->close();
$conn->close();
// 重定向回主页面
header("Location: index.php");
exit();
}
?>
步骤6:添加一些CSS(可选)
在同一文件夹中创建一个styles.css文件来为你的应用程序设置样式:
body {
font-family: Arial, sans-serif;
background-color: #f9f9f9;
color: #333;
margin: 0;
padding: 0;
}
.container {
width: 50%;
margin: 50px auto;
background: #fff;
padding: 20px;
box-shadow: 0 0 10px rgba(0, 0, 0, 0.1);
border-radius: 8px;
}
h1 {
text-align: center;
}
form {
display: flex;
justify-content: space-between;
margin-bottom: 20px;
}
form input {
flex: 1;
padding: 10px;
margin-right: 10px;
border: 1px solid #ccc;
border-radius: 4px;
}
form button {
padding: 10px 20px;
background-color: #28a745;
color: white;
border: none;
border-radius: 4px;
cursor: pointer;
}
form button:hover {
background-color: #218838;
}
ul {
list-style-type: none;
padding: 0;
}
ul li {
display: flex;
justify-content: space-between;
padding: 10px;
border-bottom: 1px solid #ddd;
}
ul li a {
color: #dc3545;
text-decoration: none;
}
步骤7:运行你的应用程序
- 打开浏览器,访问http://localhost/todo_app/index.php。
- 添加一些任务、查看任务并删除它们。🎉
恭喜!你刚刚使用PHP和MySQL构建了你的第一个数据库驱动的Web应用程序。这个简单的项目为创建更复杂的应用程序奠定了基础。你可以尝试添加诸如任务优先级或用户认证等功能。
如果你喜欢本教程,欢迎留言或分享给其他开发者。祝你编码愉快!🚀
Publish on 2025-01-10,Update on 2025-02-10